Not graded. So why do it?
Not graded. So why do it?
Operators
+
, -
, and *
are numeric operators. Here are the numeric operators you'll use in this course:
+ | Add, like 2 + 9 is 11 |
- | Subtract, like 2 - 9 is -7 |
* |
Multiply, like 2 * 9 is 18 |
/ | Divide, like 3 / 3 is 1 |
^ | Raise to a power, like 3 ^ 3 is 27) |
- | Unary minus, like -3 |
- 34 - 3
... is a numeric expression. That is, a calculation that works out a number.
Bee tee dubs, goats often call * a "splat." More fun to say than asterisk.
Geeks do the same thing.
Order
Check this out:
- dog = 2 + 3 * 4
- MsgBox dog
You might think that that would show 20 in the dialog. 2 + 3 is 5, and 5 * 4 is 20.
But actually, it would show 14.
That's because VBA doesn't use the operators from left to right. Instead, some operators have a higher precedence than others. VBA does *
before it does +
. So it's 3 * 4
is 12, and then 2 + 12
is 14.
If you wanted VBA to do the + first, add parentheses:
- dog = (2 + 3) * 4 is 20
Here are the numeric operators again, in precedence order:
() | VBA does anything in parens first. |
- | Unary minus, like -3 (that's negative 3) |
^ | Raise to a power, like 3 ^ 3 |
* and / |
Multiply and divide have equal priority, done left to right. |
+ and - | Add and subtract have equal priority, done left to right. |
The operators in...
- cat = 3 - 5 + 2 * 2 ^ 2 + 3
... would be done in this order...
- 3 - 5 + 2 * 2 ^ 2 + 3 becomes 3 - 5 + 2 * 4 + 3
- 3 - 5 + 2 * 4 + 3 becomes 3 - 5 + 8 + 3
- 3 - 5 + 8 + 3 becomes -2 + 8 + 3
- -2 + 8 + 3 becomes 6 + 3
- 6 + 3 becomes 9
Variables
Check this again:
- cat = 3 - 5 + 2 * 2 ^ 2 + 3
All of the values in the numeric expression are constants. 3 is always 3, 5 is always 5.
However, they could also be variables.
- sMouse = InputBox("Mice?")
- sLlama = InputBox("Llamas?")
- sCat = sMouse - 5 + sLlama * sLlama ^ 2 + sMouse
OK, silly variable names, but it would run.
What do they do?
Here are some programs. What would they output?
You can download a workbook with all of the code.
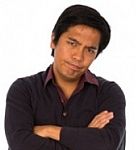
Ray
None.
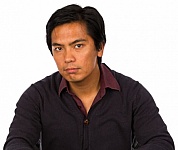
Ray
Well, I didn't make you do it. You could have just skipped the task. The reason I put the task there, is to help you learn something that might be worth knowing.
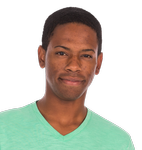
Ethan
I hear you. And you're right to question it. Is all coursework worth your time? Maybe not.
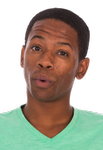
Ethan
Thanks. I think.
All I'll claim is that there's no busy work in this course. Everything's focused on the learn-so-you-can-earn-benjamins goal.
A suggestion. Give it a couple of weeks. See if you think this course is worth your time. If you think it is, don't skip the readings, or the tasks.
This isn't math
A common thing in programming:
- sAmount = InputBox("Amount?")
- aTotal = sTotal + sAmount
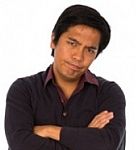
Ray
Wait, no. sTotal = sTotal + sAmount
makes no sense. A variable can't be equal to itself, plus something else. Unless sAmount
is zero.
That would be true, if this was math. But it isn't. In programming, =
means take the thing on the right, and put it in the thing on the left. Like this:
sTotal <- sTotal + sAmount
This says,
"Hey, VBA! Take sTotal
and sAmount
, and add them together. Then put the result back in sTotal
.
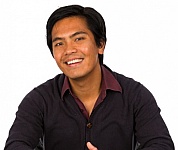
Ray
Oh, dude! I get it. It's increasing sTotal
by whatever is in sAmount
. So if sTotal
is 10, and sAmount
is 3, then sTotal
is increased by 3, to 13.
Exactly! Programs do this all the time.
An assignment statement, like sTotal = sTotal + sSales
. Adds to a variable, then puts the result back in the variable.
Numeric functions
So we have variables, like sTotal
, constants, like 72
, and operators, like *
. There's one other type of thing: functions. There are a bunch, but we'll only use a few:
Sqr() | Square root, like sqr(36) is 6 |
Abs() | Absolute value, like abs(-3.2) is 3.2 |
Rnd() | Return a random number between 0 and 1 |
For example:
- sGoat = 2
- sFish = 18
- MsgBox Sqr(sGoat * sFish)
That would show 6.
Try these
Some more tasks. They don't count towards your grade, but will help you learn.