Log in
If you're a student, please log in, so you can get the most from this page.
Programming is frustrating! For you, me, everyone. When you learn more, you're just frustrated by different things. The frustration doesn't go away.
Tell me about it! I'm always butting heads with code. These horns were straight before I started coding! Now look at them! All bent up!
Let's talk about some ways to make things easier. Easy is good!
You already learned about Option Explicit
. That helps. Here are some other things.
Incremental testing
When you have lots of code to test, bugs could hide anywhere. The solution? Don't have lots of code to test!
Write code in baby steps. Write a few lines, test, write a few more, test. When you find a bug, it will probably be in the last few lines you wrote.
Do this from now on.
Write a little code, then test it. Write a little more code, then test it.
Meaningful variable names
Check this out.
- x36 = ph3t3 + ph3t3 + y33a
Does that code look right?
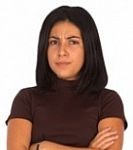
Adela
Who knows? x36
? y33a
? Could mean anything.
How about this code?
- sTotalPrice = sPrice + sPrice + sSalesTaxRate
Does that code look right?
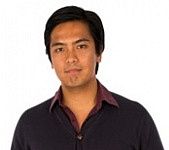
Ray
Well, no. Looks like the second + should be a *. It should be:
- sTotalPrice = sPrice + sPrice * sSalesTaxRate
Right. Because you know how sales tax works, you see the problem.
Using meaningful variable names makes your code easier to write, easier to read, and easier to fix. From now on, make sure you use meaningful variable names for all of your exercises.
Hungarian naming
Also, let's use Hungarian naming for variables. The first character tells you about the data type, like s
for Single
variables. As you learn about more data types, you'll see different characters. Knowing the types will help a lot when it comes to validation.
After the data type letter, use one or more words. The first character of each word is uppercase, like sGoatHappinessLevel
.
Use names that explain what the variable is. Long names are OK. For example, instead of sGtHap
, use sGoatHappinessLevel
.
We've already been doing this, and it seemed natural. "Total price" is sTotalPrice
. "Sales tax rate" is sSalesTaxRate
. Price is just sPrice
. You knew what these things were, even though you didn't know the Hungarian naming standard.
Ctrl+Space, your superfriend
You don't have to type in the full name of a variable when you're writing code. Suppose you have a variable called sAverage
, and want to output it into a cell. You type the first few characters of the variable name:
Then hit Ctrl+Space.
You'll see a list of the symbols that start with those characters.
There are several things that start with the letters you typed. The one you want is the third in the list. Use the down arrow to choose the one you want.
Press Tab. VBE will finish the variable name for you.
Faster, and fewer spelling errors. M0000t!
Indentation
Later, we'll talk about if statements and loops. Still, here's some code that should make sense.
In retail, some buyers are exempt from state sales tax. Maybe churches, in some states. Some items are exempt from sales tax, like food.
- sBasePrice = sUnitPrice * sNumberUnits
- If tExempt = "yes" then
- sTotalPrice = sBasePrice
- Else
- sTotalPrice = sBasePrice + sBasePrice * sSalesTaxRate
- End If
Lines 3 and 5 depend on line 2. If the buyer or the product is tax exempt, line 3 runs. Otherwise line 5 runs. Only one of them runs.
Lines 3 and 5 are indented, that is, pushed to the right (press the Tab key in VBE to indent). That makes it easy to see their execution depends on another line. Maybe they'll run, maybe they won't. Depends.
Here's the same code without the indenting.
- sBasePrice = sUnitPrice * sNumberUnits
- If tExempt = "yes" then
- sTotalPrice = sBasePrice
- Else
- sTotalPrice = sBasePrice + sBasePrice * sSalesTaxRate
- End If
It runs the same. VBA doesn't care about indenting. But it's harder for us humans to work out what's going on. That means more bugs, more time, and more frustration.
So, indent your code.
Secluded Cells
We just talked about this one. Cells
should be by itself, on one side of =
. Makes code easier to understand, and easy to change.
Comments
Some code:
- sVolume = 3.14159 * r * r * h / 4
Does that code look right?
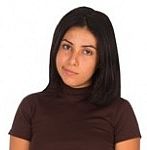
Adela
It would be easier to tell if we knew what the formula was for.
Fair enough. Let me add a comment.
- ' Compute volume of a cone.
- sVolume = 3.14159 * r * r * h / 4
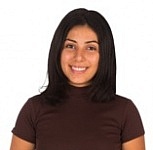
Adela
Now we know what to google. Let me check... No, the last 4 should be a 3.
You're right!
Comments are notes for people. Computers ignore them. Comments explain what code is supposed to do. They're helpful when debugging programs, or changing what the programs do.
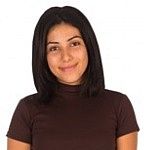
Adela
Changing what they do?
Yes. Business changes all the time, so the programs that help businesses run have to change as well. Most programmers spend more time changing existing code, than writing new programs.
The easier code is for programmers to understand, the faster and more accurate they can work. Comments are a big help.
The harder it is for you to figure something out, the more important comments are. If it was hard for you when writing the code, it will be hard for someone else to understand what you did. Comments make it easier.
Truth goat: Comments are your friends.
True dat, TG. True dat.
Avoiding bugs
We've talked about:
- Using
Option Explicit
- Incremental testing
- Meaningful variable names
- Hungarian naming
- Ctrl+Space
- Indenting
- Comments