We've seen the flag validation pattern in VBA. Here it is again, with some notes:
- tIsInputOk = "yes" Flag starts off saying that the data is OK
- 'Validate meal cost
- tUncheckedUserInput = Cells(1, 2)
- If Not IsNumeric(tUncheckedUserInput) Then
- 'Not numeric, show error.
- Cells(1, 3) = "Sorry, meal cost must be a number."
- Cells(1, 3).Font.Color = vbRed
- tIsInputOk = "no" Error, change the flag
- Else
- 'Is numeric.
- sMealCost = tUncheckedUserInput
- If sMealCost < 0 Then
- Cells(1, 3) = "Sorry, meal cost cannot be negative."
- Cells(1, 3).Font.Color = vbRed
- tIsInputOk = "no" Error, change the flag
- End If
- End If
- 'Validate tip rate
- tUncheckedUserInput = Cells(2, 2)
- If Not IsNumeric(tUncheckedUserInput) Then
- 'Not numeric, show error.
- Cells(2, 3) = "Sorry, tip rate must be a number."
- Cells(2, 3).Font.Color = vbRed
- tIsInputOk = "no" Error, change the flag
- Else
- 'Is numeric.
- sTipRate = tUncheckedUserInput
- If sTpRate < 0 Then
- Cells(2, 3) = "Sorry, tip rate cannot be negative."
- Cells(2, 3).Font.Color = vbRed
- tIsInputOk = "no" Error, change the flag
- End If
- End If
- 'Did anything go wrong?
- If tIsInputOk = "no" Then Test flag after all validation
- End
- End If
The pattern works in other languages, too. They have variables, if statements, etc. The code works the same. It just looks different.
Here's some code from commercial software that's on the market. The language is C#. Match the C# code with the VBA. I added some notes, the KRM things. They're not part of the code. I won't explain all the C# stuff, just enough, so you can see the match to VBA.
tFlourishingSlugIn
and flourishingInteractionControllerIn
are input variables. Don't worry about what they mean.
- // Validate.
- KRM: C#'s version of a @DIM@, for the flag variable.
- KRM: @bool@ is a data type that's only true or false.
- bool bIsDataOk = true;
- // Need a flourishing slug.
- KRM: Normalizing. @CleanKey@ trims, and converts to lowercase.
- _tFlourishingSlug = Utilities.CleanKey(tFlourishingSlugIn);
- if (String.IsNullOrEmpty(_tFlourishingSlug))
- {
- KRM: writes an error message to a file, rather than a cell in a worksheet.
- Utilities.LogError(
- $"FlourishingDetectableController.Setup for {this.gameObject.name}: " +
- $"tFlourishingSlugIn MT"
- );
- KRM: What does this line do?
- bIsDataOk = false;
- }
- // Must be an interaction controller.
- if (flourishingInteractionControllerIn == null)
- {
- Utilities.LogError(
- $"FlourishingDetectableController.Setup for {this.gameObject.name}: " +
- $"flourishingInteractionControllerIn MT"
- );
- bIsDataOk = false;
- }
- // Test validation flag.
- if (!bIsDataOk)
- {
- KRM: Here, @return@ works like @End@.
- return;
- }
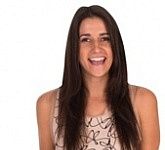
Georgina
Wow, that's so cool! Does the same thing work in Python?
Yes. It has variables, if statements, and such. (Python is used for lots of things, especially data analysis.)
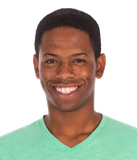
Ethan
Javascript?
Yes. (JS runs on web pages. For example, the table of contents tree on the left, with the click-to-expand-and-collapse, is written in JS.)
Java, PHP, Go, Ruby... the validation flag pattern works in all.
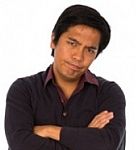
Ray
Dude, that C# code looks really complex.
It's professional level. Like, full-time-software-engineer-with-years-of-experience level. Here's the thing, though. Could you see how it does roughly the same job as the VBA?
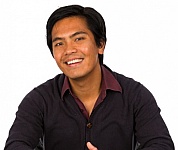
Ray
Yeah, I can see how it matches up.
Right! You're a beginner, but the ideas you're working with are used throughout programming, at all levels.
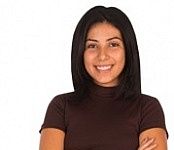
Adela
OK, so, if I wanted to learn how to write programs that run in web pages, in JavaScript. Would it be easier for me, since I've learned VBA?
Yes. You'd still have a lot to learn, but it'd be easier than it would have been. You already know patterns that work in JavaScript.
Something that annoyed me the other day. I filled in a web form by copy-and-paste. Validation code, written in JavaScript, told me the data was invalid. It wasn't. It just had an extra space at the beginning.
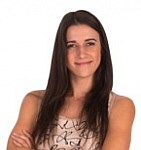
Georgina
The programmer should have trimmed the input before testing it, like we learned.
Right! JS has a trim function called, er, trim()
. I wondered, if the programmer missed that, what else might be wrong?
You know better. Trimming those spaces is good practice, no matter the language.