Not graded. So why do it?
Not graded. So why do it?
A nested If
is an If
inside another If
. This is very common.
Old people and prune juice
Some older people have trouble with..., er, their digestive processes. A dance club keeps prune juice for them, in a quiet room in the back.
- Dim sAge As Single
- sAge = InputBox("How old are you?")
- If sAge >= 21 Then
- MsgBox "Welcome! Come on in. Bring your money."
- ' Test for geezers.
- If sAge >= 50 Then
- MsgBox "There's prune juice in a quite room in the back."
- End If
- Else
- MsgBox "Sorry, you're not allowed in yet."
- End If
The geezer test is inside the can-enter-club test. You can put as much code as you want into an If
, including other If
s. The inner If
is called a nested If
.
Line 8 runs if the test in line 7 is true. Of course, line 7 runs only if the test in line 4 is true.
Line 11 runs if the test in line 4 if false.
Indenting is very important with nested If
s, otherwise it's hard to tell what goes with what.
The code...
- MsgBox "There's prune juice in a quite room in the back."
... is indented twice, because it's inside an If
, that's inside another If
.
Thanks to ZamboBambo, a former student
Here's the code again.
- Dim sAge As Single
- sAge = InputBox("How old are you?")
- If sAge >= 21 Then
- MsgBox "Welcome! Come on in. Bring your money."
- ' Test for geezers.
- If sAge >= 50 Then
- MsgBox "There's prune juice in a quite room in the back."
- End If
- Else
- MsgBox "Sorry, you're not allowed in yet."
- End If
How many message boxes would someone who is 34 see (not including the InputBox)?
How many message boxes would someone who is 74 see (not including the InputBox)?
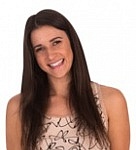
Georgina
Someone who's 34 would just see one.
- If sAge >= 21 Then True for sAge is 34.
- MsgBox "Welcome! Come on in. Bring your money." Show this.
- ' Test for geezers.
- If sAge >= 50 Then Skip...
- MsgBox "There's prune juice in a quite room in the back."
- End If ... to here.
- Else
Someone who's 74 would see two.
- If sAge >= 21 Then True for sAge is 74.
- MsgBox "Welcome! Come on in. Bring your money." Show this.
- ' Test for geezers.
- If sAge >= 50 Then Also true for sAge is 74.
- MsgBox "There's prune juice in a quite room in the back." Show this.
- End If
- Else
Great! You got it.
Your debugger friend
Code with If
statements can get complex. It's easy to make mistakes. You'll get lost, and frustrated.
The debugger is your friend here. It's critical that you learn to use it well.
Let's practice with this code. Here it is again:
- Dim sAge As Single
- sAge = InputBox("How old are you?")
- If sAge >= 21 Then
- MsgBox "Welcome! Come on in. Bring your money."
- ' Test for geezers.
- If sAge >= 50 Then
- MsgBox "There's prune juice in a quite room in the back."
- End If
- Else
- MsgBox "Sorry, you're not allowed in yet."
- End If
Make a worksheet with it. You'll need a button. I'll wait. While I'm waiting, I'll watch some of Cthulhu's goats. You can watch it, too. It's, like, homework. Yeah, that's it.
Here's how I set up the editor.
I put a breakpoint on one of the lines. You click in the grey area to make one.
What does a breakpoint do?
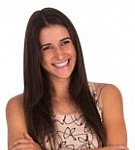
Georgina
The computer stops running the program there. You can check out the variables.
Right!
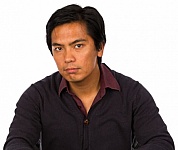
Ray
Hey, wait up. I don't see that window at the bottom.
Turn it on in the menu, with Views | Locals window.
Now, run the program. It will keep running until it hits the breakpoint.
When you ran the program, what's the first thing that you saw? The breakpoint, or something else? Why that?
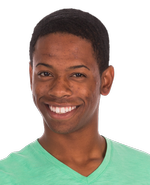
Ethan
Well, the first thing I saw was the dialog asking my age.
The line that showed that is:
- sAge = InputBox("How old are you?")
That line is before the breakpoint, so I see it first.
Right!
OK, put in an age. I just typed 33 (OK, I'm a lot older than that, I know). This is the next thing I saw:
VBA stops on the breakpoint, before it's run the highlighted line. You can see what memory looks like at that point.
Now, press F8. That runs one line. Keep pressing it, and watch as your program runs.
You can see what statements VBA runs, and what it skips. For example, I saw this:
sAge >= 50
is false. So when I pressed F8 (that is, ran the next line):
VBA skipped over the MsgBox
, to the End If
.
I pressed F8 again, and saw:
Why skip to the End If
? sAge >= 21
was true, so it ran the statements inside the If
, then skipped the statements inside the Else
.
Exercise
Birthday party planning
Cthulhu like to throw birthday parties for his goatty friends. Help him figure out how many invitations to send.
Your workbook should start off like this:
The user fills in the age and friends cells, and clicks the button. The program shows the number of invitations. Like this:
The number of invitations is the number of friends times two. (Each friend brings a pal.)
For goats 10 years old or older, the number of invitations must be at least 20. So if an old goat has 2 friends, change the number of invitations to 20.
Here's some more sample output.
Hint: nested if.
Upload your worksheet. The usual coding standards apply.